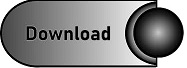
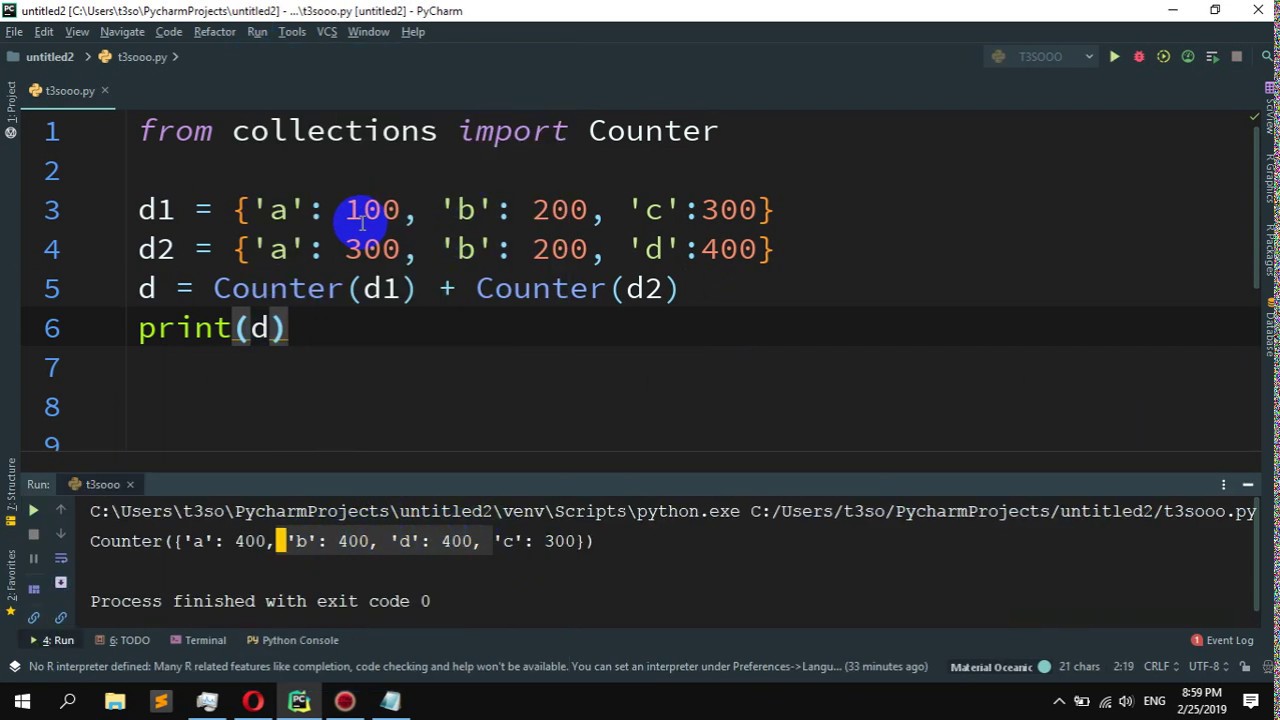
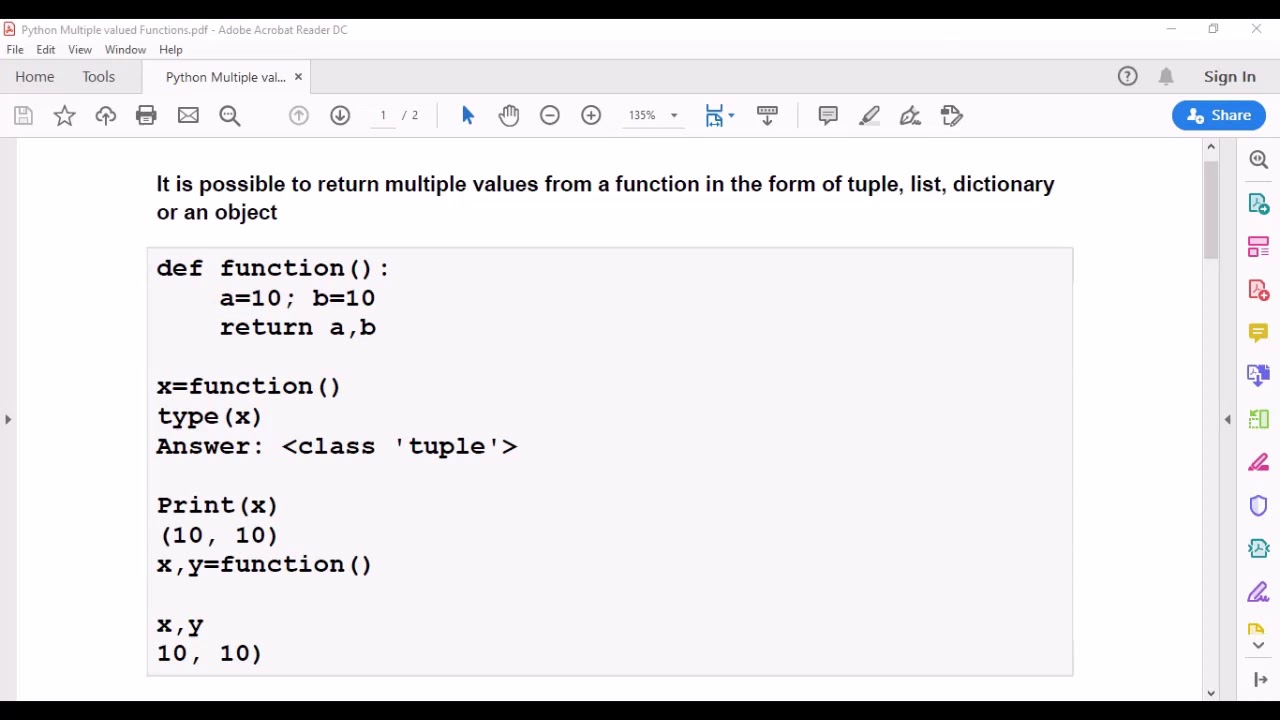
#PYTHON MERGE DICTIONARIES WITH DICTS AS VALUES CODE#
Also note that our code is general: it makes no presumptions about the dictionaries’ keys - they need not be strings nor of any other particular type. This, along with a good docstring, makes our code easy to read, understand, and debug. Note the use of simple and descriptive variables (e.g. we use the variable name key when we iterate over the keys of a dictionary). Parameters - dict1 : Dict dict2 : Dict Returns - Dict The merged dictionary """ merged = dict ( dict1 ) for key in dict2 : if key not in merged or dict2 > merged : merged = dict2 return merged Thus calling this function will mutate (change) the state of dict1, as demonstrated here:ĭef simple_merge_max_mappings ( dict1, dict2 ): """ Merges two dictionaries based on the largest value in a given mapping. Recall that dictionaries are mutable objects and that the statement merged = dict1 simply assigns a variable that references dict1 rather than creating a new copy of the dictionary. The problem with our function is that we inadvertently merge dict2 into dict1, rather than merging the two dictionaries into a new dictionary.
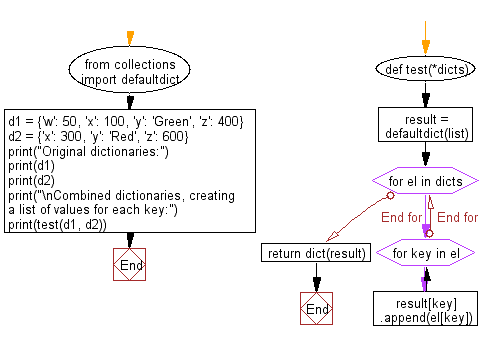
Merged is initialized to have the same mappings as dict1, this is a correct algorithm for merging our two dictionaries based on max-value. We then set a key-value from dict2 mapping in merged if that key doesn’t exist in merged or if the value is larger than the one stored in existing mapping. Thus for key in dict2 loops over every key in dict2. Recall that iterating over a dictionary will produce each of its keys one-by-one. The mergenesteddicts function recursively merges the inner dictionaries, updating the values for common keys and adding new key-value pairs. Let’s first see what this function does right. New Unpacking Operator for Merging Dictionaries in Python 3. The constructor creates a new dictionary containing the merged keys and values. Def buggy_merge_max_mappings ( dict1, dict2 ): # create the output dictionary, which contains all # the mappings from `dict1` merged = dict1 # populate `merged` with the mappings in dict2 if: # - the key doesn't exist in `merged` # - the value in dict2 is larger for key in dict2 : if key not in merged or dict2 > merged : merged = dict2 return merged The dict () Constructor: The dict () constructor can be used to merge dictionaries by passing the dictionaries to be merged as arguments to the constructor.
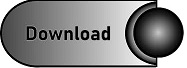